T3
These images are generated like the Mandelbrot set fractals for the
iterative sequence Z[0]=0 and z[n+1]<-z[n]^4+c. The colour at a point c
represents the number of iterations necessary for the system to reach a value from which
we know the sequence diverges to infinity. The edge of the set is tracked
by computing the variance the colours in a 16x24 tile, and then
a random choice is made amongst the seven tiles with highest variance.
The iteration program is written in C, and the A.I. features (edge
detection) are written in APL. The Image Magick package does conversions
from .XPM format to .gif format.
tri000.gif | 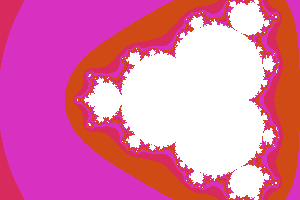 |
|
tri001.gif | 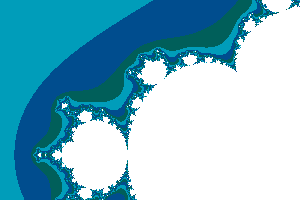 |
|
tri002.gif | 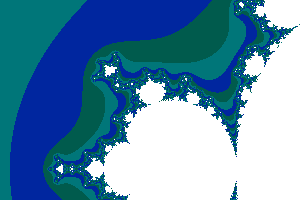 |
|
tri003.gif | 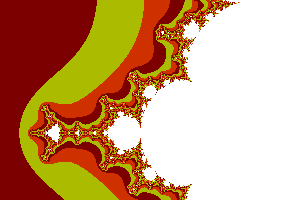 |
|
tri004.gif | 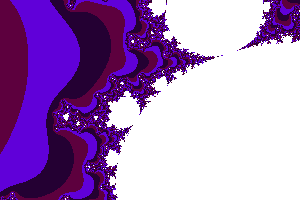 |
|
tri005.gif | 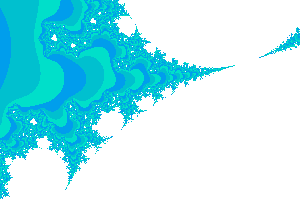 |
|
tri006.gif | 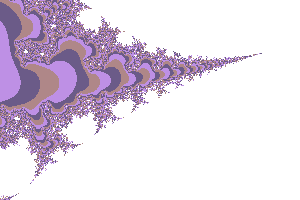 |
|
tri007.gif | 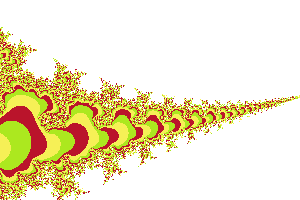 |
|
tri008.gif | 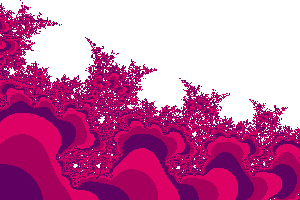 |
|
tri009.gif | 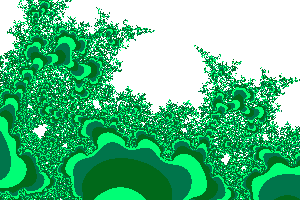 |
|
tri010.gif | 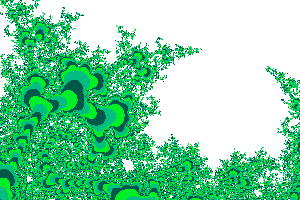 |
|
tri011.gif | 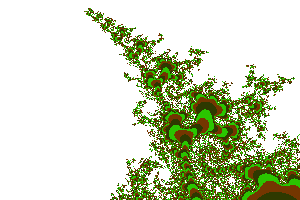 |
|
tri012.gif | 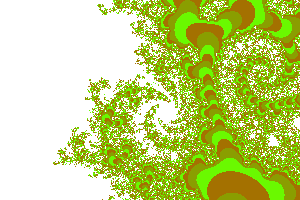 |
|
tri013.gif | 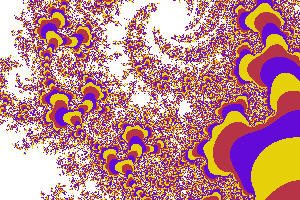 |
|
tri014.gif | 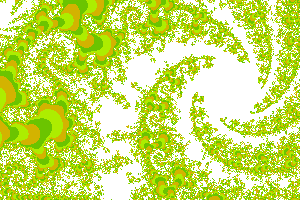 |
|
tri015.gif | 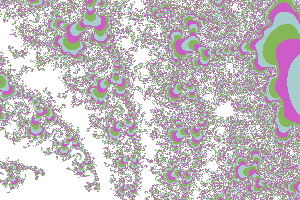 |
|
tri016.gif | 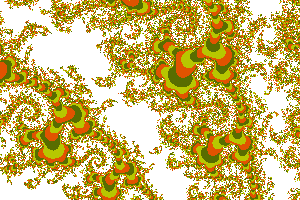 |
|
tri017.gif | 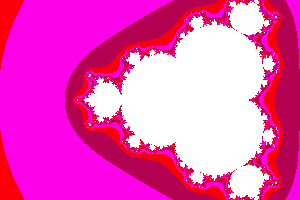 |
|
tri018.gif | 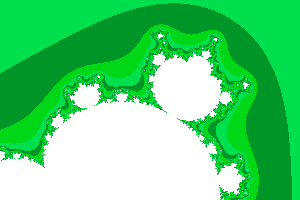 |
|
tri019.gif | 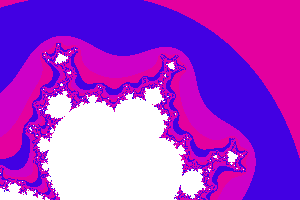 |
|
tri020.gif | 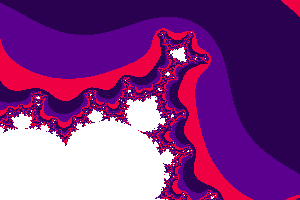 |
|
tri021.gif | 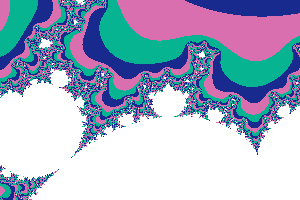 |
|
tri022.gif | 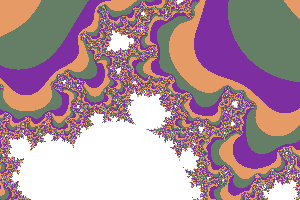 |
|
tri023.gif | 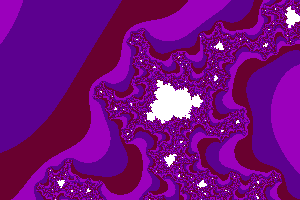 |
|
tri024.gif | 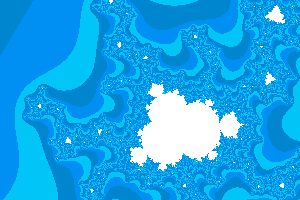 |
|
tri025.gif | 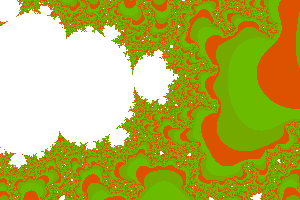 |
|
tri026.gif | 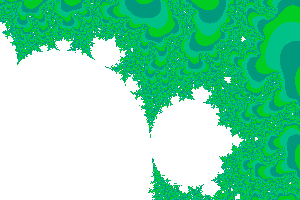 |
|
tri027.gif | 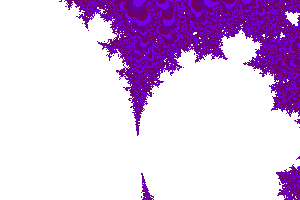 |
|
Back to the Top
/* mandelbrot tile
mt -zone y0 x0 y1 x1 -size ny nx -ofile file
minimum memory varsion. Allocate only one line
*/
#include
#include
#define ERROR -1
double zw[4] = {-2,-2,2,2};
int size[2] = {24,80};
int kf = 0;
double eps = 1.0e-18; /* -eps epsilon */
int bailout = 35; /* maximum iteration */
char *ofile = NULL;
char *lut = ".123456789ABCDEF";
int nlut;
int j_orbit(), j3_orbit(), j4_orbit(), j5_orbit(), j6_orbit();
int (*func[])() = {j_orbit, j3_orbit, j4_orbit, j5_orbit, j6_orbit};
main(argc, argv)
int argc;
char **argv;
{
extern double atof();
extern char *malloc();
FILE *ofp = NULL;
char *s;
char *rup, *t;
int i, j, k;
double x, y, dx, dy;
for(argv++, argc--; 0 < argc--; argv++) {
s = *argv;
if (s[0] == '-') switch(s[1]) {
case 'z': /* zone */
zw[0] = atof(*++argv);
zw[1] = atof(*++argv);
zw[2] = atof(*++argv);
zw[3] = atof(*++argv);
argc -= 4;
break;
case 's': /* size */
size[0] = atoi(*++argv);
size[1] = atoi(*++argv);
argc -=2;
break;
case 'l': /* lut */
lut = *++argv;
argc--;
break;
case 'o': /* ofile */
ofile = *++argv;
argc--;
break;
case 'b': /* -bailout */
bailout = atoi(*++argv);
argc--;
break;
case 'e': /* -eps */
eps = atof(*++argv);
argc--;
break;
case 'f': /* -function */
kf = atoi(*++argv);
argc--;
break;
default: break;
}
}
nlut = strlen(lut);
if (ofile) {
ofp = fopen(ofile, "w");
if (ofp == NULL) return ERROR;
}
else ofp = stdout;
fprintf(ofp, "-size %d %d -zone %f %f %f %f\n",
size[0], size[1], zw[0], zw[1], zw[2], zw[3]);
if (NULL == (rup = malloc(1+size[1]))) return ERROR;
dy=(zw[2]-zw[0])/(size[0]-1);
dx=(zw[3]-zw[1])/(size[1]-1);
for (i=0, y=zw[0]; i < size[0]; i++, y +=dy) {
for (t=rup, j=0, x=zw[1]; j < size[1]; j++, x += dx) {
/* k =j_orbit(y, x); */
k = (func[kf])(y,x);
*t++ = (k == 0) ? ' ': lut[k % nlut];
}
for (t = rup, j=0; j < size[1]; j++) fputc(*t++, ofp);
fputc('\n', ofp);
}
if (ofile) fclose (ofp);
return 0;
}
/* dynamic system z<-z^2+c with global parameters eps, bailout */
int j_orbit(cy, cx)
double cy, cx;
{
double x = 0.0, y = 0.0;
double x1, y1, x2, y2;
int j;
for(j = 0; j < bailout; j++) {
x2= x*x; y2=y*y;
if (4.1 < x2+y2) return j;
x1 = cx + x2-y2;
y1 = cy + 2*x*y;
x = x1;
y = y1;
}
return 0;
}
/* dynamic system z<-z^3+c */
int j3_orbit(cy, cx)
double cy, cx;
{
double x = 0.0, y = 0.0;
double x1, y1, x2, y2;
int j;
for(j = 0; j < bailout; j++) {
x2= x*x; y2=y*y;
if (4.1 < x2+y2) return j;
x1 = cx + x*(x2-3*y2);
y1 = cy + y*(3*x2-y2);
x = x1;
y = y1;
}
return 0;
}
/* dynamic system z<-z^4+c */
int j4_orbit(cy, cx)
double cy, cx;
{
double x = 0.0, y = 0.0;
double x1, y1, x2, y2;
int j;
for(j = 0; j < bailout; j++) {
x2= x*x; y2=y*y;
if (4.1 < x2+y2) return j;
x1 = cx + x2*x2+y2*y2-6*x2*y2;
y1 = cy + 4*x*y*(x2-y2);
x = x1;
y = y1;
}
return 0;
}
/* dynamic system z<-z^5+c */
int j5_orbit(cy, cx)
double cy, cx;
{
double x = 0.0, y = 0.0;
double x1, y1, x2, y2, x2y2, x4, y4;
int j;
for(j = 0; j < bailout; j++) {
x2= x*x; y2=y*y; x2y2=x2*y2; x4=x2*x2; y4=y2*y2;
if (4.1 < x2+y2) return j;
x1 = cx + x*(x2*x2+5*y2*y2-10*x2y2);
y1 = cy + y*(5*x4-10*x2y2+y4);
x = x1;
y = y1;
}
return 0;
}
/* dynamic system z<-z^6+c */
int j6_orbit(cy, cx)
double cy, cx;
{
double x = 0.0, y = 0.0;
double x1, y1, x2, y2, x2y2, x4, y4;
int j;
for(j = 0; j < bailout; j++) {
x2= x*x; y2=y*y; x2y2=x2*y2; x4=x2*x2; y4=y2*y2;
if (4.1 < x2+y2) return j;
x1 = cx + x2*x2*(x2-16*y2)+y2*y2*(15*x2-y2);
y1 = cy + x*y*(6*(x4+y4)-20*x2y2);
x = x1;
y = y1;
}
return 0;
}